How to convert Buffer data to JSON in Node.js?
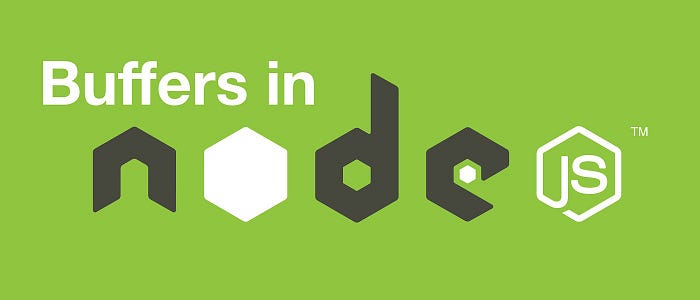
Converting a buffet to JSON in JavaScript can be a useful skill to have, as JSON is a widely used format for data exchange between web applications. In this blog, we’ll explore how to convert a JavaScript object to a JSON string using the built-in JSON.stringify()
method.
To convert a Buffer to JSON, you can use the
toJSON()
method in the Buffer.
//Syntax for Converting Json Object to Buffer
const json = buff.toJSON();
Let’s start with an example object:
const buffet = {
name: "All You Can Eat",
cuisine: "International",
dishes: [
{
name: "Sushi",
type: "Japanese",
price: 20.99
},
{
name: "Pizza",
type: "Italian",
price: 15.99
},
{
name: "Tacos",
type: undefined,
price: 10.99
}
]
};
Here, we have an object that represents a buffet, with properties for the buffet name, cuisine, and an array of dishes, each with a name, type, and price.
To convert this object to a JSON string, we can call the JSON.stringify()
method and pass in our object as an argument:
const json = JSON.stringify(buffet);
console.log(json);
The JSON.stringify()
method will return a string that represents our object in JSON format:
//Output
{
"name": "All You Can Eat",
"cuisine": "International",
"dishes": [
{
"name": "Sushi",
"type": "Japanese",
"price": 20.99
},
{
"name": "Pizza",
"type": "Italian",
"price": 15.99
},
{
"name": "Tacos",
"price": 10.99
}
]
}
Explaination
Note that the JSON.stringify()
method automatically converts any properties with values that are not valid JSON (such as functions or undefined values) to null
.
Converting an object to a JSON string is a common task in web development, as JSON is often used to exchange data between web applications. By using the built-in JSON.stringify()
method in JavaScript, you can easily convert an object to a JSON string and send it to a server or use it in your application.
In the above example, we can see the price of “Tacos” removed automatically as it’s undefined
See this example live in Codepen.io
This skill can be useful in web development and other areas where JSON is used for data exchange. By mastering this technique, you can effectively handle and manipulate data in a web application, allowing you to create more robust and dynamic applications.
Thanks for reading. If you have thoughts on this, be sure to leave a comment. Also, if you found this article helpful, give me some claps.